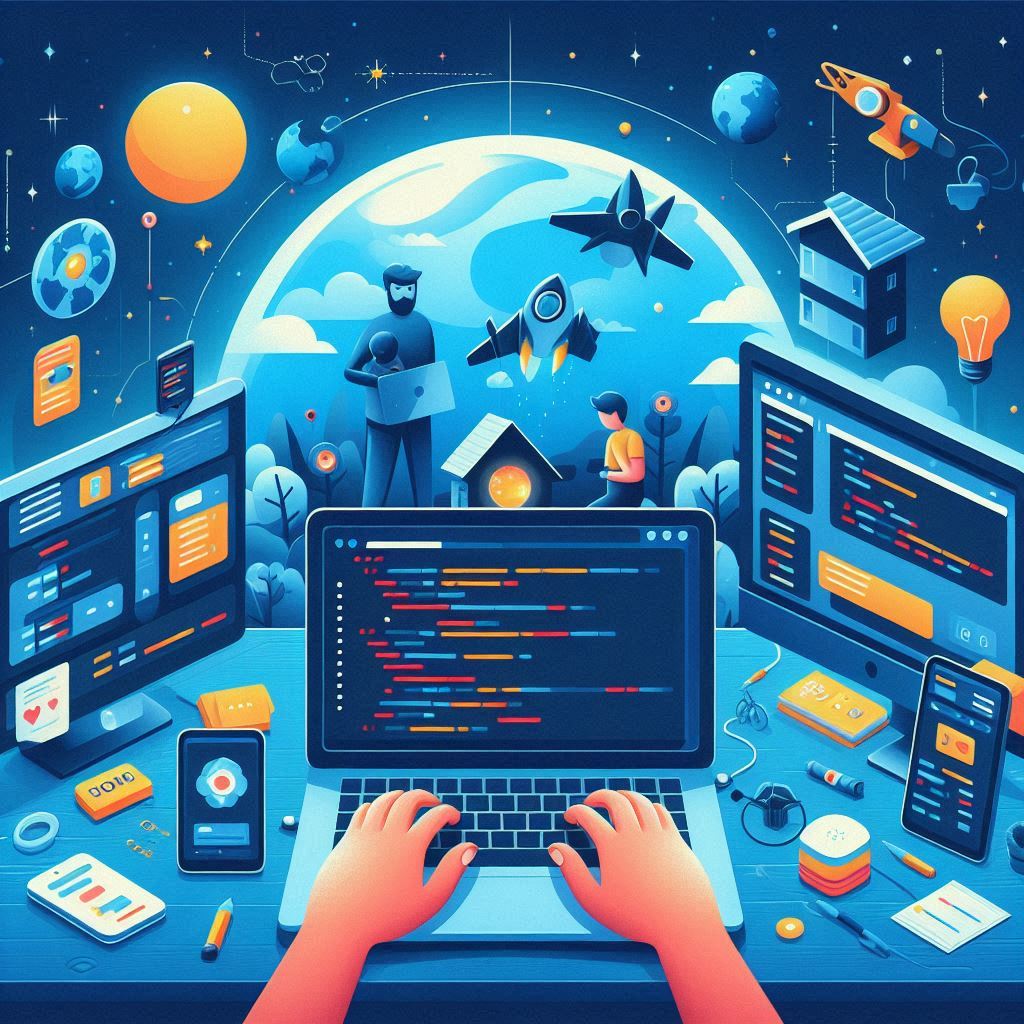
Building a website using WordPress is one of the best platforms due to versatility and huge ecosystem. WordPress has plugin which is one of the basic building blocks of customizable aspect. Developers can create and add custom functionality to a WordPress website without touching any code due to the WordPress plugin system. In this tutorial, we will take you through the entire process of Build a Plugin for WordPress, from setting up your environment to publishing your own plugin.
1. Introduction
Why Build a Plugin for WordPress journey?
WordPress plugins allow you to safely adjust WordPress to fulfill specific needs, for either personal needs or client requests. Custom plugins enable you to incorporate elements such as contact forms, SEO optimization tools, or even individualized integration functionalities.
Prerequisites
To get started, you’ll need a basic understanding of:
- PHP: WordPress plugins are primarily built using PHP.
- WordPress Coding Standards: These ensure your code is clean and maintainable.
- File Management: Familiarity with organizing and editing files on a server.
2. Setting Up Your Development Environment
Before build a plugin for WordPress, prepare your tools:
- Code Editor: Use tools like Visual Studio Code or Sublime Text for efficient coding.
- Local Server: Install XAMPP, Local by Flywheel, or similar software to create a local development environment.
- WordPress Installation: Set up a local WordPress site for testing
- Debugging: Enable debugging in
wp-config.php
by settingdefine( 'WP_DEBUG', true );
3. Understanding the Anatomy of a Plugin
Plugin Structure
let’s build a plugin for WordPress. A WordPress plugin typically includes:
- Main PHP File: Contains the core functionality and the plugin header.
- Optional Subdirectories:
/assets
: For images, CSS, or JavaScript files./includes
: For modular PHP code./languages
: For translation files.
Plugin Header
The header is a comment block that contains information about the plugin. For example:
<?php
/*
Plugin Name: My First Plugin
Description: A simple plugin example.
Version: 1.0
Author: Your Name
*/
4. Creating Your First Plugin
Building your first WordPress plugin is a straightforward process. Here’s a detailed breakdown:
Step 1: Create a Plugin Folder
Navigate to your WordPress installation directory, then to the wp-content/plugins/
directory. Create a new folder for your plugin. For this example, let’s call it my-first-plugin
. This folder will hold all the files related to your plugin.
Step 2: Add the Main PHP File
Inside the newly created folder, create a file named my-first-plugin.php
. Open this file in your code editor and add the required plugin header. The header provides basic information about your plugin.
<?php
/*
Plugin Name: My First Plugin
Plugin URI: https://example.com/
Description: A simple plugin to demonstrate WordPress plugin creation. from article how to build a plugin for WordPress
Version: 1.0
Author: Your Name
Author URI: https://example.com/
License: GPL2
*/
Step 3: Add Functionality
For example, let’s add a simple footer message:
add_action( 'wp_footer', function() {
echo '<p style="text-align:center;">Hello from My First Plugin!</p>';
} );
Step 4: Activate the Plugin
Go to your WordPress Admin Dashboard → Plugins. You will see your new plugin listed. Click “Activate” to enable it. Visit your website to see the footer message added by your plugin.
5. Adding Core Features
Core functionality makes a plugin useful and attractive. WordPress offers many ways to extend its functionality using hooks, filters and shortcodes. Below are some detailed examples to serve as inspiration for developing your own plugin:
Hooks and Filters
Hooks allow you to interact with WordPress’s actions and filters.
Add a Custom Header to All Posts
You can prepend custom content to every post using a filter:
add_filter( 'the_content', function( $content ) {
if ( is_single() ) {
$custom_header = '<h2 style="color: blue;">Welcome to My Blog</h2>';
return $custom_header . $content;
}
return $content;
} );
Redirect Users on Login
Customize where users land after logging in:
add_filter( 'login_redirect', function( $redirect_to, $requested_redirect_to, $user ) {
if ( in_array( 'subscriber', (array) $user->roles ) ) {
return home_url( '/subscriber-dashboard' );
}
return $redirect_to;
}, 10, 3 );
Shortcodes
Shortcodes make it easy to insert dynamic content anywhere on your site.
Create a Button Shortcode
Add a shortcode to display a styled button:
add_shortcode( 'custom_button', function( $atts ) {
$atts = shortcode_atts(
[ 'text' => 'Click Me', 'url' => '#' ],
$atts
);
return '<a href="' . esc_url( $atts['url'] ) . '" class="custom-button" style="padding:10px 20px; background:#0073aa; color:white; text-decoration:none; border-radius:5px;">' . esc_html( $atts['text'] ) . '</a>';
} );
Use the shortcode [custom_button text="Learn More" url="https://example.com"]
to generate the button.
how is going your build a plugin for WordPress?
List Recent Posts
Display recent posts dynamically:
add_shortcode( 'recent_posts', function() {
$recent_posts = wp_get_recent_posts( [ 'numberposts' => 5, 'post_status' => 'publish' ] );
$output = '<ul>';
foreach ( $recent_posts as $post ) {
$output .= '<li><a href="' . get_permalink( $post['ID'] ) . '">' . esc_html( $post['post_title'] ) . '</a></li>';
}
$output .= '</ul>';
return $output;
} );
Enqueueing Styles and Scripts
Adding custom CSS or JavaScript files improves the user experience.
Add a Frontend Script
Ensure your script loads only on the frontend:
add_action( 'wp_enqueue_scripts', function() {
wp_enqueue_script(
'my-plugin-script',
plugins_url( '/assets/js/my-script.js', __FILE__ ),
[ 'jquery' ],
'1.0',
true
);
} );
Add an Admin-Only Script
Use admin hooks to load scripts exclusively in the WordPress admin area:
add_action( 'admin_enqueue_scripts', function() {
wp_enqueue_script(
'my-plugin-admin-script',
plugins_url( '/assets/js/admin-script.js', __FILE__ ),
[],
'1.0',
true
);
} );
Article: The Benefits of Displaying Panorama Images on Your Website and How to Do It
6. Advanced Functionality
Creating Custom Admin Pages
You can add settings pages for your plugin using the add_menu_page()
function.
add_action( 'admin_menu', function() {
add_menu_page(
'My Plugin Settings', // Page title
'My Plugin', // Menu title
'manage_options', // Capability
'my-plugin-settings', // Menu slug
function() { // Callback function
echo '<h1>My Plugin Settings</h1>';
echo '<form method="post">Settings form content here from build a plugin for WordPress</form>';
}
);
} );
Database Interaction
For custom data storage, interact with the WordPress database using $wpdb
Example: Save Data to a Custom Tablephp
global $wpdb;
$table_name = $wpdb->prefix . 'custom_table';
// Insert Data
$wpdb->insert( $table_name, [ 'column_name' => 'value' ] );
// Fetch Data
$results = $wpdb->get_results( "SELECT * FROM $table_name" );
Integrating Third-Party APIs
Use APIs to fetch external data and display it on your site.
Example: Fetch Data from a Public API
add_shortcode( 'weather', function() {
$response = wp_remote_get( 'https://api.example.com/weather' );
if ( is_wp_error( $response ) ) {
return 'Unable to fetch weather data.';
}
$data = wp_remote_retrieve_body( $response );
return '<p>Weather Data: ' . esc_html( $data ) . '</p>';
} );
7. Best Practices
- Security: Use nonces, sanitize inputs, and escape outputs to prevent vulnerabilities.
- Performance: Load assets conditionally and minimize resource usage.
- Coding Standards: Follow WordPress coding standards.
8. Testing and Debugging
- Use tools like Query Monitor and WP Debug Log for debugging.
- Test your plugin in multiple environments: local, staging, and live servers.
- Check compatibility with other plugins and themes.
9. Packaging and Releasing Your Plugin
Preparing for Release
- Include a
readme.txt
file with plugin details. - Organize files logically and document your code.
Submitting to the WordPress Plugin Repository
- Create an account on WordPress.org.
- Submit your plugin and follow the guidelines for approval.
if you like this article ‘How to build a plugin for WordPress’, please share with your friends who also want to learn plugin development.